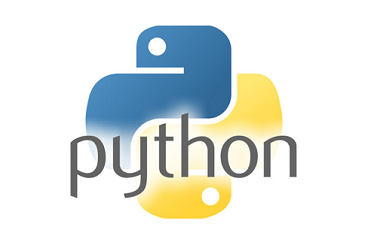
2 字符串的使用
2.1 使用规则
– Python申明字符串可使用单引号,例如(‘…’)
– Python申明字符串可使用双引号,例如(“…”)
– Python使用“\”作为转义字符
– 使用单引号如果使用单引号,需要使用转义字符,使用双引号可省略
– 使用双引号如果使用双引号,需要使用转义字符,使用单引号可省略
2.2 实操理解
# python Python 2.7.5 (default, Aug 4 2017, 00:39:18) [GCC 4.8.5 20150623 (Red Hat 4.8.5-16)] on linux2 Type "help", "copyright", "credits" or "license" for more information. >>> 'spam eggs' 'spam eggs' >>> "sapm eggs" 'sapm eggs' >>> 'doesn\'t' "doesn't" >>> '"Yes," he said.' '"Yes," he said.' >>> "doesn't" "doesn't" >>> "\"yes,\" he said." '"yes," he said.' >>>
3 禁用字符串输出的转义
3.1 使用规则
– print函数可在第一个引号前加“r”来禁用转义
3.2 实操理解
# python Python 2.7.5 (default, Aug 4 2017, 00:39:18) [GCC 4.8.5 20150623 (Red Hat 4.8.5-16)] on linux2 Type "help", "copyright", "credits" or "license" for more information. >>> print 'C:\some\name' C:\some ame >>> print r'C:\some\name' C:\some\name >>>
4 多行输出和逻辑行
4.1 使用规则
– Python的多行输出可使用三重引号实现,如”””…”””或”’…”’
– Python的逻辑行也可使用“\”符号实现
4.2 实操理解
# python Python 2.7.5 (default, Aug 4 2017, 00:39:18) [GCC 4.8.5 20150623 (Red Hat 4.8.5-16)] on linux2 Type "help", "copyright", "credits" or "license" for more information. >>> print """Hello, ... World!""" Hello, World! >>> print "Hello, \ ... World!" Hello, World! >>>
5 重复输出与字符串连接
5.1 使用规则
– Python使用“*”操作符加数字可实现字符串重复输出
– Python使用“+”操作符实现字符串的连接
– 相邻的量子字符串会自动连接
5.2 实操理解
# python Python 2.7.5 (default, Aug 4 2017, 00:39:18) [GCC 4.8.5 20150623 (Red Hat 4.8.5-16)] on linux2 Type "help", "copyright", "credits" or "license" for more information. >>> # 3 times 'un', followed by 'ium' ... 3 * 'un' + 'ium' 'unununium' >>> 'un' * 3 + 'ium' 'unununium' >>> 'Py' 'thon' 'Python' >>> text = ('Put serveral strings within parentheses' ... 'to have then joined together.') >>> text 'Put serveral strings within parenthesesto have then joined together.'
带变量的连接错误的示范:
# python Python 2.7.5 (default, Aug 4 2017, 00:39:18) [GCC 4.8.5 20150623 (Red Hat 4.8.5-16)] on linux2 Type "help", "copyright", "credits" or "license" for more information. >>> prefix = 'Py' >>> prefix 'thon' # can't concatenate a variable and a string literal File "", line 1 prefix 'thon' # can't concatenate a variable and a string literal ^ SyntaxError: invalid syntax >>> ('un'*3) 'ium' File "", line 1 ('un'*3) 'ium' ^ SyntaxError: invalid syntax >>>
带变量的连接正确的示范:
# python Python 2.7.5 (default, Aug 4 2017, 00:39:18) [GCC 4.8.5 20150623 (Red Hat 4.8.5-16)] on linux2 Type "help", "copyright", "credits" or "license" for more information. >>> prefix = 'Py' >>> prefix + 'thon' 'Python'
6 索引的使用
6.1 使用规则
– Python字符串实质是存储到字符串中
– Python字符串的字符可使用下标索引访问
– Python字符串的下标支持反向访问(负数表示)
– 负索引的“-0”等于“0”,所以索引是从“-1”开始
6.2 实操理解
# python Python 2.7.5 (default, Aug 4 2017, 00:39:18) [GCC 4.8.5 20150623 (Red Hat 4.8.5-16)] on linux2 Type "help", "copyright", "credits" or "license" for more information. >>> word = 'Python' >>> word[0] 'P' >>> word[1] 'y' >>> word[2] 't' >>> word[3] 'h' >>> word[4] 'o' >>> word[5] 'n' >>> word[-1] 'n' >>> word[-2] 'o' >>> word[-3] 'h' >>> word[-4] 't' >>> word[-5] 'y' >>> word[-6] 'P' >>>
7 索引切片
7.1 Python的原文解析
– Python可以使用“:”操作符前后加索引数字指定索引的区间(i:i),进行字符串的截取
– “:i”操作符右边带索引数字,左边为空,表示索引开始到索引i的区间
– “i:”操作符左边带索引数字示,左边为空,表示索引i的区间到索引末尾的区间
– 索引“i”的值可以为负值
7.2 实操理解
# python Python 2.7.5 (default, Aug 4 2017, 00:39:18) [GCC 4.8.5 20150623 (Red Hat 4.8.5-16)] on linux2 Type "help", "copyright", "credits" or "license" for more information. >>> word = "Python" >>> word[0:2] 'Py' >>> word[2:6] 'thon' >>> word[:2] 'Py' >>> word[2:] 'thon' >>> word[:2] + word[2:] 'Python' >>> word[:-2] 'Pyth' >>> word[-2:] 'on' >>> word[:-2] + word[-2:] 'Python'
8 索引视图
8.1 索引视图的模型
+---+---+---+---+---+---+ | P | y | t | h | o | n | +---+---+---+---+---+---+ 0 1 2 3 4 5 6 -6 -5 -4 -3 -2 -1
8.2 模型的规则
– 第一行使用数字0到6标记出索引的位置
– 第二行使用数字相应的负数标记出索引的位置
– 假设切片的起点为i与终点为j,那么i与j所代表的索引数字标识出的区间就是切片后的字符串
– 切片长度是i与j的差(非负索引,且i与j都在边界内)
– 使用超出范围的索引会导致报错
– 使用超出范围的切片索引会被自动纠正(优雅地自动纠正)
8.3 实操理解
# python Python 2.7.5 (default, Aug 4 2017, 00:39:18) [GCC 4.8.5 20150623 (Red Hat 4.8.5-16)] on linux2 Type "help", "copyright", "credits" or "license" for more information. >>> word = "Python" >>> word[42] Traceback (most recent call last): File "", line 1, in IndexError: string index out of range >>> word[4:42] 'on' >>> word[42:] ''
9 错误的字符串赋值与引用
9.1 使用规则
– Python字符串可以整串赋值
– Python字符串赋值后,每个索引代表的单独字符变量不能再次单独赋值
– Python被赋值的字符串可以用于创建新的字符串
9.2 实操理解
# python Python 2.7.5 (default, Aug 4 2017, 00:39:18) [GCC 4.8.5 20150623 (Red Hat 4.8.5-16)] on linux2 Type "help", "copyright", "credits" or "license" for more information. >>> word = "Python" >>> word[0] 'P' >>> word[0]='J' Traceback (most recent call last): File "", line 1, in TypeError: 'str' object does not support item assignment >>> word[:2] 'Py' >>> word[:2] = 'py' Traceback (most recent call last): File "", line 1, in TypeError: 'str' object does not support item assignment >>> 'J' + word[1:] 'Jython' >>>
10 内置函数的使用
10.1 使用规则
– 可使用内置函数“len()”返回字符串的长度
10.2 实操理解
# python Python 2.7.5 (default, Aug 4 2017, 00:39:18) [GCC 4.8.5 20150623 (Red Hat 4.8.5-16)] on linux2 Type "help", "copyright", "credits" or "license" for more information. >>> s='supercalifragilisticexpialidocious' >>> len(s) 34 >>>
参阅文档:
==================================================
https://docs.python.org/2.7/tutorial/index.html
https://docs.python.org/2.7/
没有评论